Overview
This chapter describes how to use the Color Camera SDK, and includes code snippets from the sample application.
C++ Sample Application
The Desktop Windows Color Camera SDK Sample Application shows how to call the Camera SDK methods, configure the MP7 Camera and receive image data.
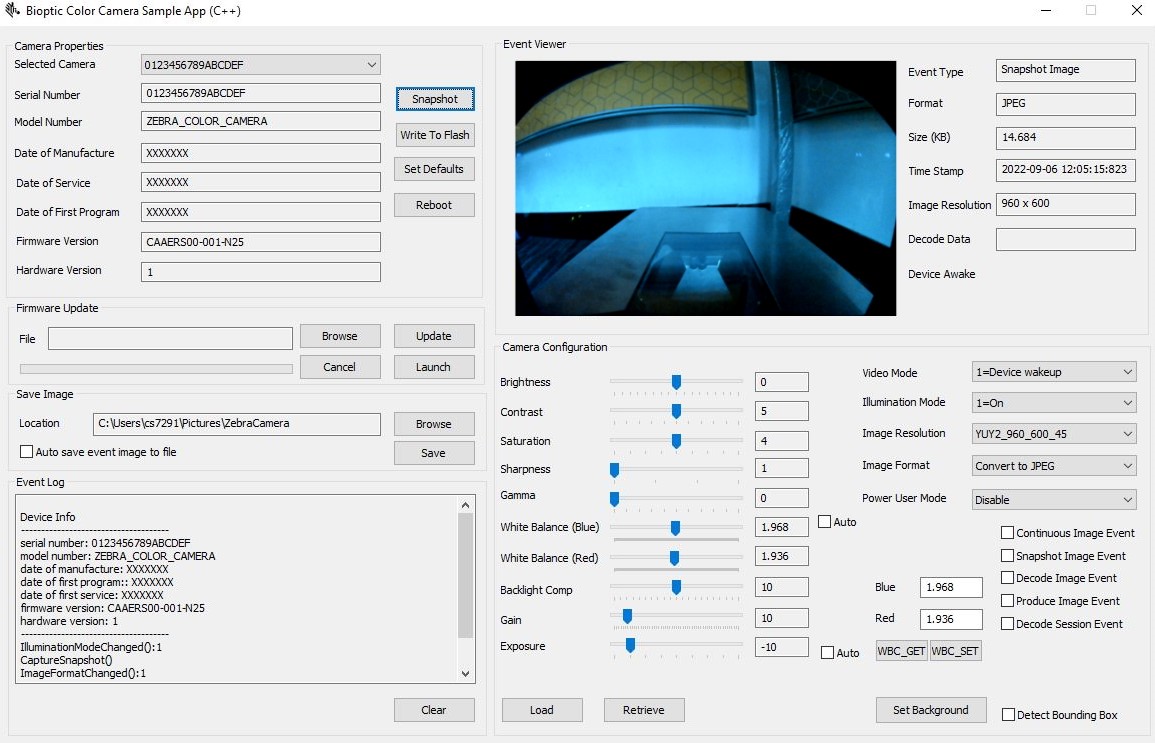
C# Sample Application
The Color Camera SDK C# sample application that uses COM wrapper DLL (camera_sdk.dll) is shown below.
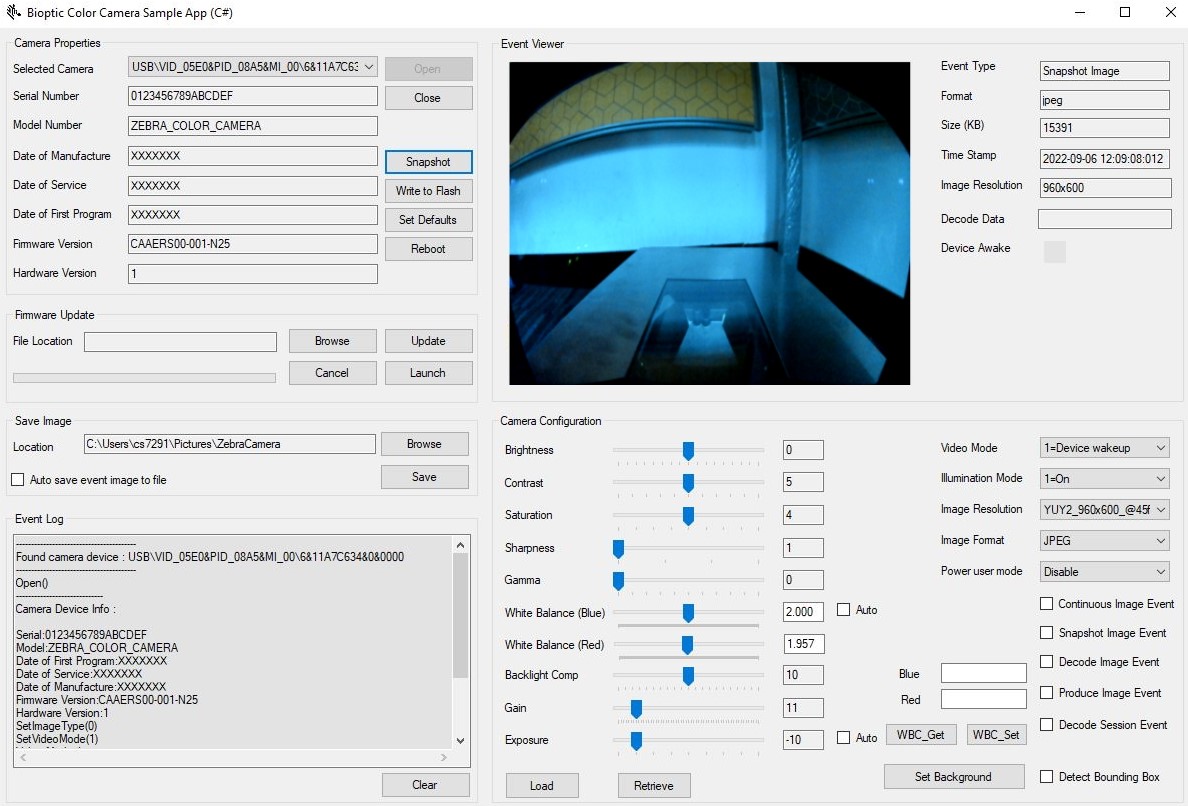
White Balance Component
White Balance Component value is a double value with range 0 to 4.000. Camera SDK presents this value in fixed point representation with a 16-bit value, 12 bits allocated to floating points.
The min, max and default value returned from the SDK may not be correct for the white balance component property. Correct Values for those properties are:
Min – 0
Max – 16384 (0x4000)
Resolution - 1
To receive the default value of White Balance Component Values set all settings to defaults by calling SetDefaults() method on the camera and then retrieve the White Balance Component Values.
Below sample code illustrate how to use read and write WhiteBalanceComponent values using camera SDK.
C++ API
#define FLOATING_POINT 12
/**
* Converts fixed point value to a double.
*/
double WBFixedToDouble(uint16_t val)
{
return ((double)val / (double)(1 << FLOATING_POINT));
}
/**
* Converts double to a fixed pont value.
*/
uint16_t WBDoubleToFixed(double val)
{
return (uint16_t)(val * (1 << FLOATING_POINT));
}
/**
* Read white balance component value from the camera.
*/
void GetWhiteBalance(std::shared_ptr camera)
{
int blue = 0, red = 0;
blue = camera->WhiteBalanceComponent.Value().Blue();
red = camera->WhiteBalanceComponent.Value().Red();
}
/**
* Write white balance component values to the camera.
*/
Void SetWhiteBalance(std::shared_ptr camera)
{
double blue = 1.5;
double red = 2.5;
uint16_t shourt_blue, short_red;
shourt_blue = WBDoubleToFixed(blue);
short_red = WBDoubleToFixed(red);
WhiteBalance Wb((int)shourt_blue, (int)short_red);
camera->WhiteBalanceComponent.Value(Wb);
}
Camera SDK COM Wrapper (C# API)
#define FLOATING_POINT 12
/**
* Converts fixed point value to a double.
*/
double WBFixedToDouble(uint16_t val)
{
return ((double)val / (double)(1 << FLOATING_POINT));
}
/**
* Converts double to a fixed pont value.
*/
uint16_t WBDoubleToFixed(double val)
{
return (uint16_t)(val * (1 << FLOATING_POINT));
}
/**
* Read white balance component value from the camera.
*/
void GetWhiteBalance(std::shared_ptr camera)
{
int blue = 0, red = 0;
blue = camera->WhiteBalanceComponent.Value().Blue();
red = camera->WhiteBalanceComponent.Value().Red();
}
/**
* Write white balance component values to the camera.
*/
Void SetWhiteBalance(std::shared_ptr camera)
{
double blue = 1.5;
double red = 2.5;
uint16_t shourt_blue, short_red;
shourt_blue = WBDoubleToFixed(blue);
short_red = WBDoubleToFixed(red);
WhiteBalance Wb((int)shourt_blue, (int)short_red);
camera->WhiteBalanceComponent.Value(Wb);
}
In Camera SDK COM wrapper, White Balance Component values are encoded in to single 32bit integer. The high order bits contains the blue component value (16-bit) and low order bits contains the red component value (16-bit), represented in fixed point representation. See C#/C++ Sample Application section (sample app sources) for more details.
/// <summary>
/// Converts 4.12 fixed point format to double
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
double WBToDouble(int value)
{
var val_ = (double)value / (double)(1 << 12);
return val_;
}
/// <summary>
/// Converts double to 4.12 fixed point format.
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
int DoubleToWB(double value)
{
var val_ = (int)(value * (1 << 12));
return val_;
}
/// <summary>
/// Splits 32 bit combined white balance components value to 16 bit white balance component
/// blue and 16 bit white balance component red value.
/// </summary>
/// <param name="propertyValue">32bit white balance component property value.</param>
/// <param name="blueValue">16 bit white balance component blue value.</param>
/// <param name="redValue">16 bit white balance component red value.</param>
void GetWhiteBalanceComponentValues(int propertyValue, out int blueValue, out int redValue)
{
blueValue = propertyValue >> 16 & 0x0000FFFF;
redValue = propertyValue & 0x0000FFFF;
}
/**
* Read white balance component value from the camera.
*/
private void GetWhiteBalance(Camera camera)
{
int propertyValue = -1;
int isAutoEnabled = False;
int blueValue = 0;
int redValue = False;
camera.GetWhiteBalance(out propertyValue, out isAutoEnabled);
GetWhiteBalanceComponentValues(propertyValue, out blueValue, out redValue);
Var redValueDouple = WBToDouble(redValue);
Var blueValueDouple = WBToDouble(blueValue);
}
MP7 Color Camera Power User Mode
Power User Mode Configuration Guide
The MP7 Color Camera supports contextual switching between optimized camera settings for different scenarios. This guide outlines how to modify the settings within each context to suit your needs. For details on default values and when each context takes effect, please see the Power User Mode Contexts section.
Enabling Power User ModeEnabling power user mode is done by setting a value of 0x1 to Zebra Extension control 9.
Configuring Power User ContextsConfiguring contexts is done by modifying Zebra Extension controls 2 to 5, with control 2 corresponding to Context 1. Each control contains an array of 26 bytes. The structure of the array is shown below:
uint32_t min_auto_exposure;
uint32_t max_auto_exposure;
uint32_t exposure;
uint16_t min_auto_gain;
uint16_t max_auto_gain;
uint16_t gain;
uint16_t white_balance_temp;
uint16_t white_balance_blue_gain;
uint16_t white_balance_red_gain;
uint8_t exposure_auto;
uint8_t white_balance_auto;
Ordering of bytes in the array will depend on the method used to transmit the extension unit command, but by default (such as in the supplied MP7CC_cmdline.exe) White Balance Auto will be the leftmost byte in the array and Minimum Auto Exposure will be rightmost.
For convenience, a batch file called “SetContexts.bat” demonstrates packing the bytes together and sending them to the camera. This file is included with all SDK installations.
Power User Mode Contexts
For MP7 four Power User contexts available:
Context 1: Video mode set to Device Wakeup and white LED stopped>
Defaults:
- Auto exposure off, 1 ms exposure
- 1.7x gain
- Manual white balance. 2.865 blue gain, 0.847 red gain
Context 2: Video mode set to Device Wakeup and white LED running
Defaults:
- Auto exposure on. 1ms minimum, 3ms maximum
- Auto gain on. 1.7x minimum, 20x maximum
- Auto white balance
Context 3: Video mode set to Continuous and illumination mode off
Defaults:
- Auto exposure on. 1ms minimum, 3ms maximum
- Auto gain on. 1.7x minimum, 20x maximum
- Auto white balance
Context 4: Video mode set to Continuous and illumination mode on
Defaults:
- Auto exposure on. 1ms minimum, 3ms maximum
- Auto gain on. 1.7x minimum, 20x maximum
- Manual white balance. 2.865 blue gain, 0.847 red gain