Overview
The OPOS driver does not support any I/O functions directly to the scanner. However, the OPOS sample application exposes management access to an RSM-ready scanner through the Zebra Scanner SDK. Through the DirectIO feature, a POS application communicates directly with the underlying CoreScanner Service.
Information in this chapter is applicable to both scanner and scale devices.
DirectIO Methods
Syntax
LONG DirectIO(
_In_ LONG nCommand,
_Inout_ LONG *lpData,
_Inout_ BSTR *pbstrIOXML
);
DirectIO Method Parameters:
nCommand[in]: Commands to be executed by OPOS Service. Following are the possible values for this parameter.
enum DIO_Opcodes
{
DIO_GET_SCANNERS = 1,
DIO_RSM_ATTR_GETALL = 5000,
DIO_RSM_ATTR_GET = 5001,
DIO_RSM_ATTR_GETNEXT = 5002,
DIO_RSM_ATTR_SET = 5004,
DIO_RSM_ATTR_STORE = 5005,
NCRDIO_SCAL_LIVE_WEIGHT = 604,
NCRDIO_SCAL_STATUS = 601,
NCRDIO_SCAL_DIRECT = 605
NCRDIO_SCAN_NOT_ON_FILE = 508,
NCRDIO_SCAN_RESET = 502,
NCRDIO_SCAN_STATUS = 503,
NCRDIO_SCAN_DIRECT = 507
};
lpData: Indicates the CoreScanner Status. Refer to the Scanner SDK User's guide for CoreScanner status code description
pbstrIOXML: This value holds input and output arguments for the commands in XML format.
DirectIO Commands
GET_SCANNERS: This will retrieve all the scanners connected to the system and identified by CoreScanner service. No input XML is required for this command.
OutXml: This contains the raw XML string of all the connected scanners in the format:
<scanners>
<scanner type="mode">
<scannerID>id1</scannerID>
<serialnumber>serial_number</serialnumber>
<GUID>S_N:XXXXXXXXXXXXXXXXXXXXXX</GUID>
<VID>vendor_id</VID>
<PID>product_id</PID>
<modelnumber>model_number</modelnumber>
<DoM>date_of_manufacture</DoM>
<firmware>firmware_version</firmware>
</scanner>
</scanners>
RSM_ATTR_GETALL: This will retrieve a list of supported RSM attributes of a connected device. The Product Reference Guide specified for the connected scanner contains the definitions of all these attributes.
InXml
<inArgs>
<scannerID>scanner_id</scannerID>
<!-- ScannerID of one of the scanners which could be retrieved by executing GET_SCANNERS op-code -->
</inArgs>
InOutXml: This contains the raw XML string of all the supported RSM attributes:
<outArgs>
<scannerID>scanner_id</scannerID>
<arg-xml>
<modelnumber>DS8108-XXXXXXXXXXXX</modelnumber>
<serialnumber>serial_number</serialnumber>
<GUID>S_N:XXXXXXXXXXXXXXXXXXXXXXXXXX</GUID>
<response>
<opcode>5000</opcode>
<attrib_list>
<attribute name="">0</attribute>
<attribute name="">1</attribute>
<attribute name="">2</attribute>
<attribute name="">3</attribute>
<attribute name="">4</attribute>
<attribute name="">5</attribute>
...
...
<attribute name="">25102</attribute>
<attribute name="">25103</attribute>
<attribute name="">25104</attribute>
<attribute name="">25105</attribute>
</attrib_list>
</response>
</arg-xml>
</outArgs>
The value of each attribute in the returned XML list can be accessed using the RSM_ATTR_GET command.
NOTE: Calling this is not necessary if the attributes you wish to SET, or GET are known ahead of time.
RSM_ATTR_GET: This command can be used to retrieve scanner attribute information including value, data type, and permission. For more information, refer to the Zebra Scanner SDK for Windows Developer's Guide.
InXml
<inArgs>
<scannerID>scanner_id</scannerID>
<cmdArgs>
<arg-xml>
<attrib_list>attribute1[,attribute2,…,attributeN]</attrib_list>
<!-- a list of scanner attribute numbers separated by commas -->
</arg-xml>
</cmdArgs>
</inArgs>
OutXml
<outArgs>
<scannerID>scanner_id</scannerID>
<arg-xml>
<modelnumber>DS8108-XXXXXXXXXXX</modelnumber>
<serialnumber>serial_number</serialnumber>
<GUID>XXXXXXXXXXXXXXXXXXXXX</GUID>
<response>
<opcode>5001</opcode>
<attrib_list>
<attribute>
<id>attribute1</id>
<name></name>
<datatype>F</datatype>
<permission>RWP</permission>
<value>True</value>
</attribute>
</attrib_list>
</response>
</arg-xml>
</outArgs>
RSM_ATTR_GET_NEXT: This will retrieve the value of the next available attribute of the given attribute number. Refer to the Scanner SDK documentation for input XML for this command.
InXml
<inArgs>
<scannerID>scanner_id</scannerID>
<cmdArgs>
<arg-xml>
<attrib_list>3</attrib_list>
</arg-xml>
</cmdArgs>
</inArgs>
OutXml
<outArgs>
<scannerID>scanner_id</scannerID>
<arg-xml>
<modelnumber>DS9208-XXXXXXXXXX</modelnumber>
<serialnumber>XXXXXXXXXXXX</serialnumber>
<GUID>S_N:XXXXXXXXXXXX</GUID>
<response>
<opcode>5002</opcode>
<attrib_list>
<attribute>
<id>4</id>
<name></name>
<datatype>F</datatype>
<permission>RWP</permission>
<value>True</value>
</attribute>
</attrib_list>
</response>
</arg-xml>
</outArgs>
RSM_ATTR_SET or RSM_ATTR_STORE: Sets (volatile, cleared after device reboot) or stores (non-volatile, retained after device reboot) attributes respectively, specified in the XML string given in the obj argument. No OutXml for these two commands.
InXml
<inArgs>
<scannerID>scanner_id</scannerID>
<cmdArgs>
<arg-xml>
<attrib_list>
<attribute>
<id>rsm_attribute_number</id>
<datatype>valid_datatype</datatype>
<value>value_maching_datatype</value>
</attribute>
</attrib_list>
</arg-xml>
</cmdArgs>
</inArgs>
The following are the valid values:
- rsm_attribute_number: One of the scanners accepted, writeable attributes numbers.
- valid_datatype: A valid datatype character: F, B, W, D, A, S
- value_matching_datatype: A value that is consistent with the datatype and the datatype range. For example, 'F' is a 'Boolean' value that may be either 'false' or 'true'. A 'B' datatype is 'Byte' which ranges from 0-255.
For example, to disable UPC Code 128, the actual XML string passed into the obj argument would look this:
<inArgs>
<scannerID>1</scannerID>
<cmdArgs>
<arg-xml>
<attrib_list>
<attribute>
<id>7</id>
<datatype>F</datatype>
<value>False</value>
</attribute>
</attrib_list>
</arg-xml>
</cmdArgs>
</inArgs>
NCR_DIO_SCANER_NOF: This command is used to execute the NCR “Not on File” tone pattern configured through Registry key value. The tone pattern can be set for any logical device by setting the following configuration.
NCRDIO_SCAN_RESET: This command is used to reset the device.
NCRDIO_SCAN_STATUS: This command is used to get the IBM USB status bytes of the scanner. Status bytes are given as a string.
NCRDIO_SCAN_DIRECT: This command is used to execute direct IO commands GetAll, Get, GetNext, Set and Store attributes directly without the corresponding direct IO command for these operations. Direct IO OpCode for these operations is passed as an attribute in the InXml.
InXml
<inArgs>
<scannerID>scanner_id</scannerID>
<opcode>5001</opcode>
<cmdArgs>
<arg-xml>
<attrib_list>1</attrib_list>
</arg-xml>
</cmdArgs>
</inArgs>
OutXml
<?xml version="1.0" encoding="UTF-8"?>
<outArgs>
<scannerID>scanner_id</scannerID>
<arg-xml>
<modelnumber>DS9208-XXXXXXXXXXX</modelnumber>
<serialnumber>XXXXXXXXXXXXX</serialnumber>
<GUID>S_N:XXXXXXXXXXXXXX</GUID>
<response>
<opcode>5001</opcode>
<attrib_list>
<attribute>
<id>1</id>
<name></name>
<datatype>F</datatype>
<permission>RWP</permission>
<value>True</value>
</attribute>
</attrib_list>
</response>
</arg-xml>
</outArgs>
NCR_DIO_SCAL_LIVE_WEIGHT: This command is used to get the live weight value of the scale. No need of providing an InOutXml argument.
NCRDIO_SCALE_STATUS: This command is used to get the IBM USB status bytes of the scale. Status bytes are given as a string. No need of providing an InOutXml argument.
NCRDIO_SCALE_DIRECT: This command is used to execute direct IO commands GetAll, Get, GetNext, Set and Store attribute directly without the corresponding direct IO command for these operations. Direct IO OpCode for these operations is passed as an attribute in the InXml.
InXml
<inArgs>
<scannerID>scanner_id</scannerID>
<opcode>5001</opcode>
<cmdArgs>
<arg-xml>
<attrib_list>34</attrib_list>
</arg-xml>
</cmdArgs>
</inArgs>
OutXml
<outArgs>
<scannerID>scanner_id</scannerID>
<arg-xml>
<modelnumber>DS9208-XXXXXXXXXXX</modelnumber>
<serialnumber>XXXXXXXXXXXXXXX </serialnumber>
<GUID>S_N:XXXXXXXXXXXXXXXXXXXX</GUID>
<response>
<opcode>5001</opcode>
<attrib_list>
<attribute>
<id>34</id>
<name></name>
<datatype>B</datatype>
<permission>RWP</permission>
<value>1</value>
</attribute>
</attrib_list>
</response>
</arg-xml>
</outArgs>
Return Value
If the function succeeds, the return value will be OPOS_SUCCESS. Else other relevant OPOS result codes defined in UPOS Specification will return.
Using OPOS Sample Application to send DirectIO Commands
DirectIO operations can be done using the panel highlighted in red, as shown in the screenshot given below.
The required DirectIO command can be selected from the drop-down list named as the Command.
The OutXML corresponding to the selected DirectIO command can be retrieved by clicking on the Execute button.
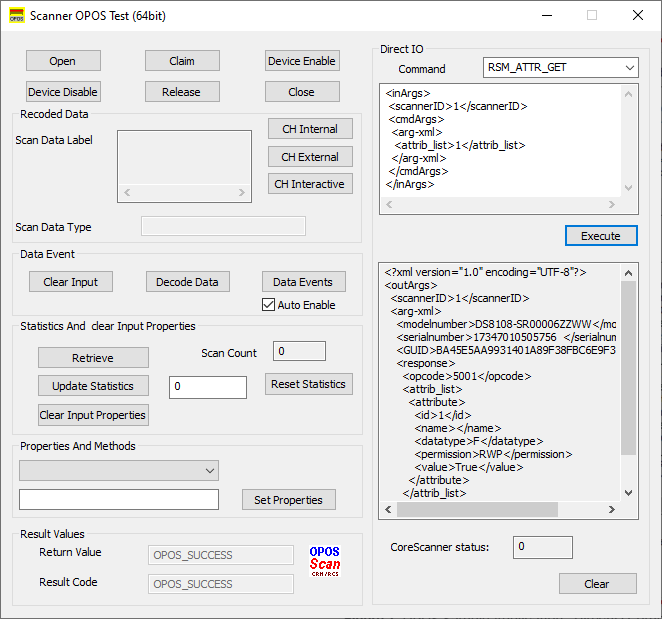
To send Direct I/O commands using the OPOS sample application:
- Connect a Zebra scanner and switch to a management-enabled host mode such as IBM OPOS, IBM Hand-held, IBM Table-top, or SNAPI.
- Open the OPOS sample application for Scanner.
- Click Open and select ZEBRA_SCANNER as the service object name.
- Click Claim.
To retrieve a list of all the scanners connected to the PC:
- Select GET_SCANNERS as DirectIO opcode from the Direct I/O section.
- Click Execute. The Direct I/O OutXml text box populates with XML with all the attached scanners.
To retrieve all attributes available in the scanner:
- Select RSM_ATTR_GETALL as DirectIO opcode from Direct I/O section.
- In the Direct I/O InXml text box, change the value of the ScannerID XML element to the scannerID from which you want to retrieve attributes. Use the GET_SCANNER command to find available ScannerIDs.
- Click Execute. The Direct I/O OutXml text box populates with XML with attribute numbers of all supported attributes.
To retrieve more information for an attribute:
- Select RSM_ATTR_GET as DirectIO opcode from the Direct I/O section.
- In the Direct I/O InXml text box, change the value of the ScannerID XML element to the required scanner ID, and enter a comma-separated list of attribute numbers as the value of attrib_list.
- Click Execute. The Direct I/O OutXml text box populates with XML containing a list of attributes with values and other attribute-related details.
To temporarily set or permanently store the value of an attribute:
- Select RSM_ATTR_SET or RSM_ATTR_STORE as DirectIO opcode from the Direct I/O section.
- In the Direct I/O InXml text box, change the value of the ScannerID XML element to the required scanner ID and change the values of data_type and the value XML elements accordingly as obtained by executing the command RSM_ATTR_GET.
- Click Execute. For these Direct I/O op-codes, an OutXml does not populate.
Upon executing each opcode, the CoreScanner Status field populates with the status of the underlying CoreScanner driver. Any value other than 0 indicates an error.