Overview
This section provides step-by-step instructions on developing Xamarin Framework based RFID applications for Android and iOS with Microsoft Visual Studio 2019.
Development Environment
Please refer the instructions provided below for configuring development environment in the respective platform.
Microsoft Windows
-
Install Visual Studio 2019 on Windows computer
https://docs.microsoft.com/en-us/visualstudio/install/install-visual-studio?view=vs-2019 -
Follow instruction in the provided link below to configure Xamarin.iOS.
https://docs.microsoft.com/en-us/xamarin/ios/get-started/installation/windows/?pivots=windows -
Additionally, follow instructions below in linking to a MAC which is mandatory requirement.
https://docs.microsoft.com/en-us/xamarin/ios/get-started/installation/windows/connecting-to-mac/
macOS
-
Follow instructions in the provided link to install Visual Studio 2019 on Mac OS. Make sure to select “iOS + Xamarin.Forms” workload during the installation.
https://docs.microsoft.com/en-us/visualstudio/mac/installation?view=vsmac-2019
Create Xamarin iOS Project
Open Visual Studio 2019, navigate to File → New Solution → iOS → Single View App and create the iOS application by following the wizard.
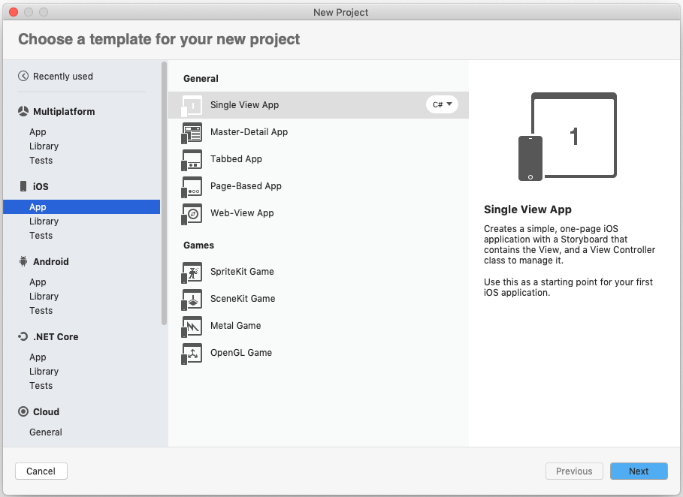
Provide an App Name and the Organization Identifier.
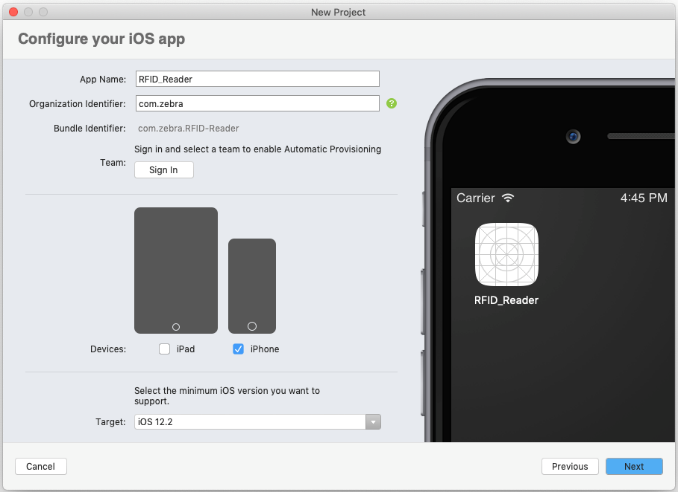
Provide a Project Name and create the project.
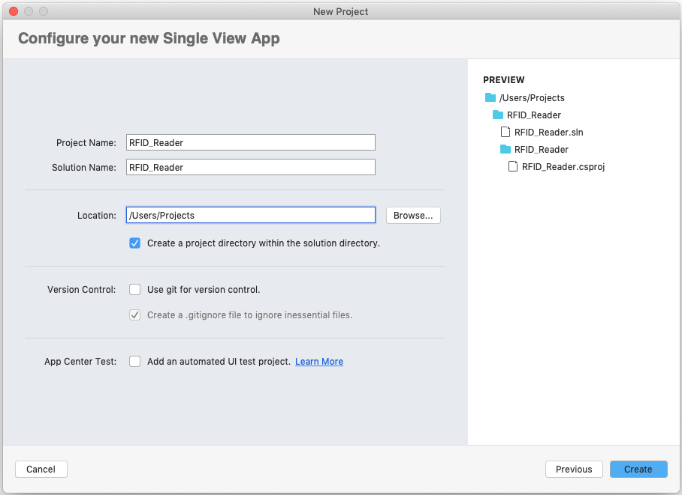
Add the 'ZebraiOSRfidLibrary.dll' to the root folder of the project.
5Then add a reference to the 'ZebraRfidSdk.dll' by right click on References → Edit References → .Net Assembly → Browse
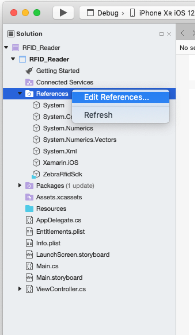
Update the 'Info.plist' as described here.
Add following entries under “Required background modes” property:
- App communicates with an accessory
- App communicates using CoreBluetooth
- App shares data using CoreBluetooth
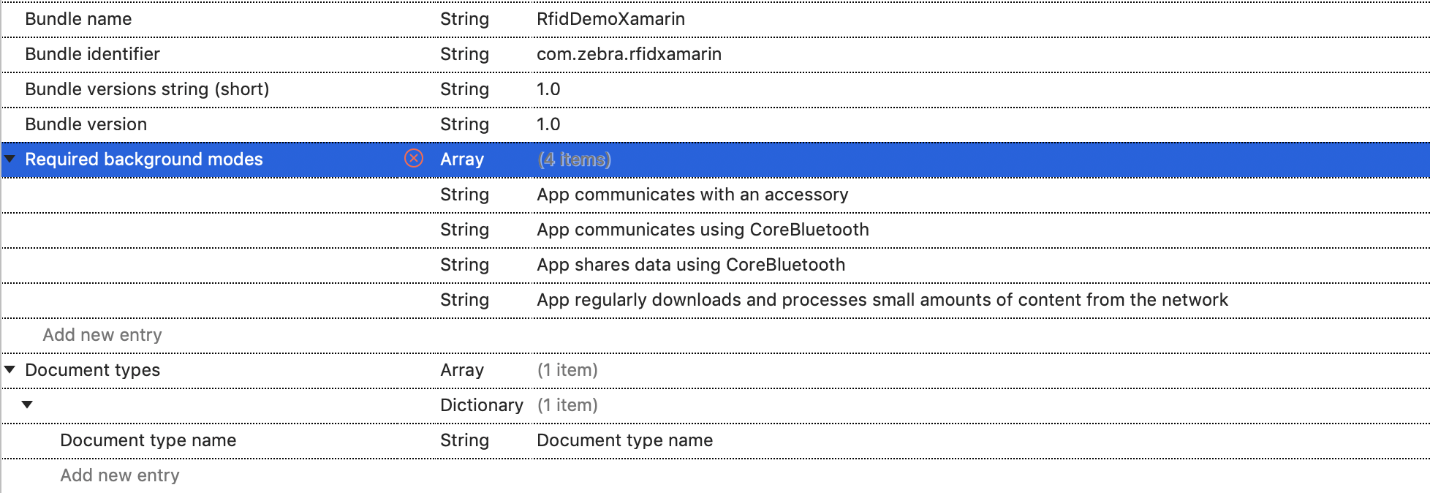
Add 'com.zebra.rfd8x00_easytext' String under 'Supported external accessory protocols' property.
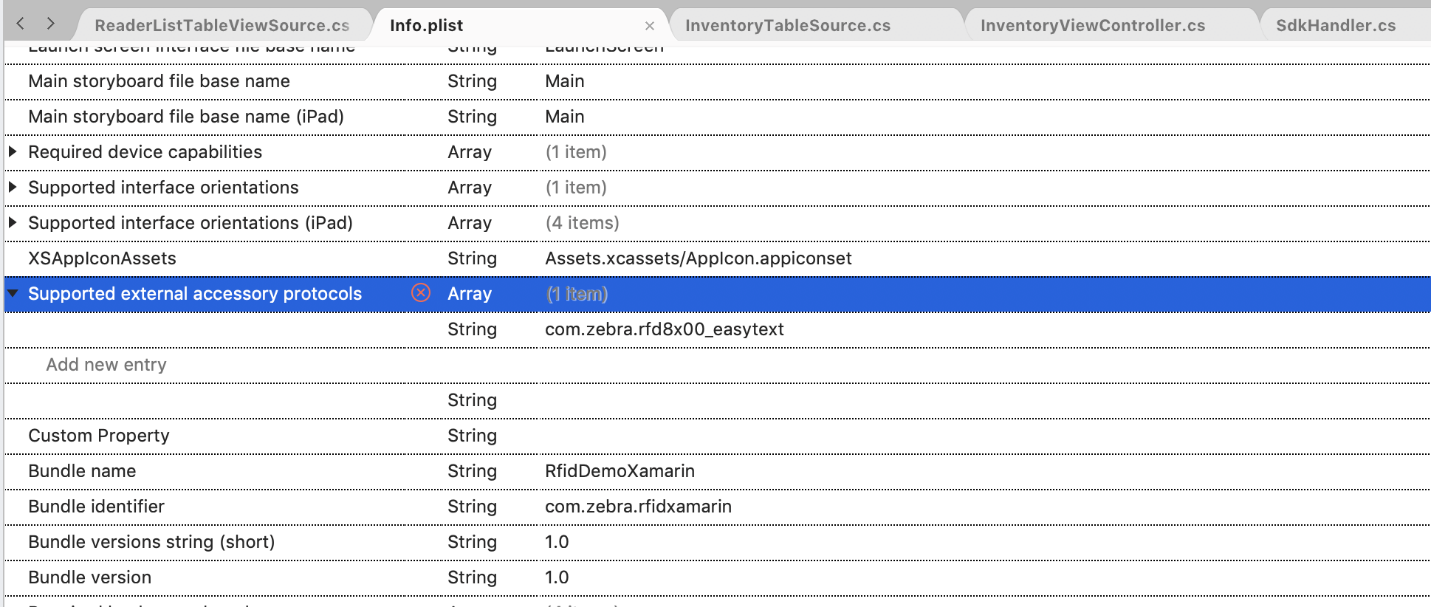
Create Xamarin Forms iOS Project
Open Visual Studio 2019 IDE, navigate to File → New Solution → Multiplatform → App → Blank Forms App and create the iOS application by following the wizard.
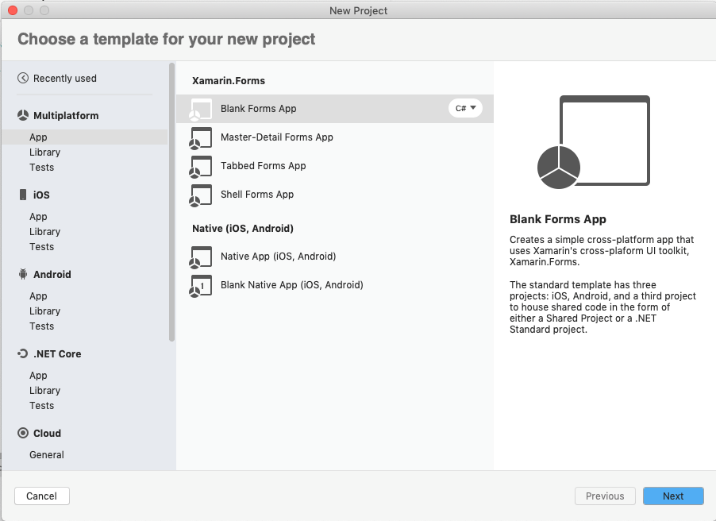
Provide an App Name and the Organization Identifier.
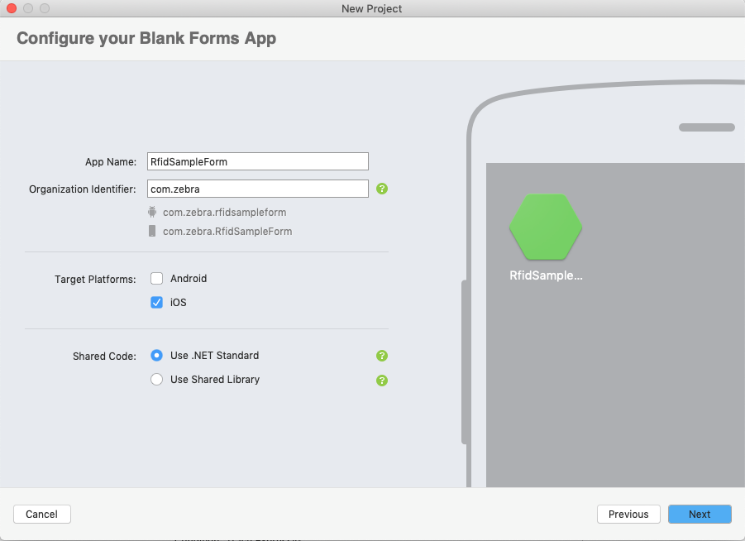
Provide a Project Name and create the project.
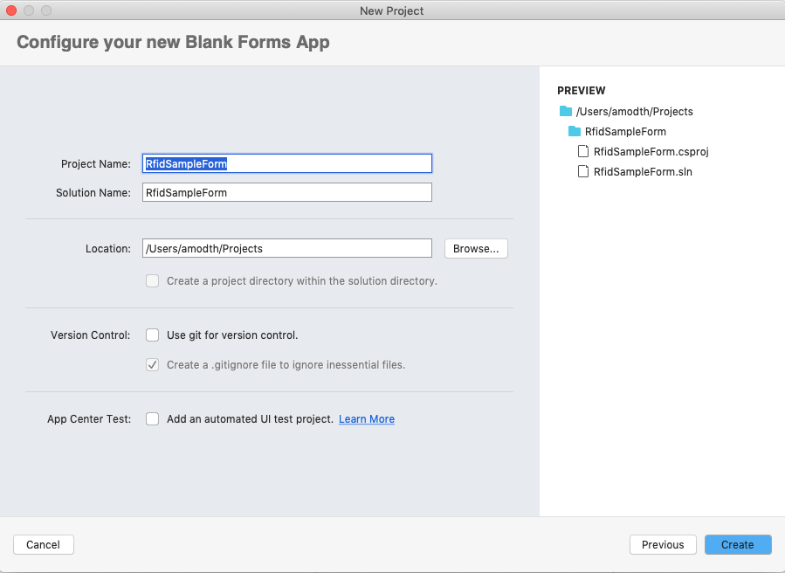
Add the 'ZebraiOSRfidLibrary.dll' to the root folder of the iOS native project.
Then add a reference to the 'ZebraRfidSdk.dll' by right click on References → Edit References → .Net Assembly → Browse.
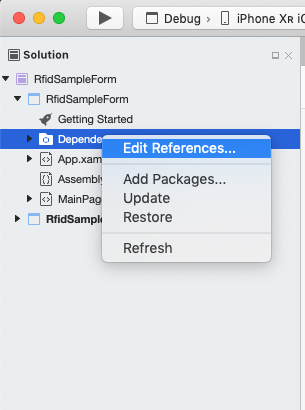
Update the 'Info.plist' as described here.
Add following entries under 'Required background modes' property:
- App communicates with an accessory
- App communicates using CoreBluetooth
- App shares data using CoreBluetooth
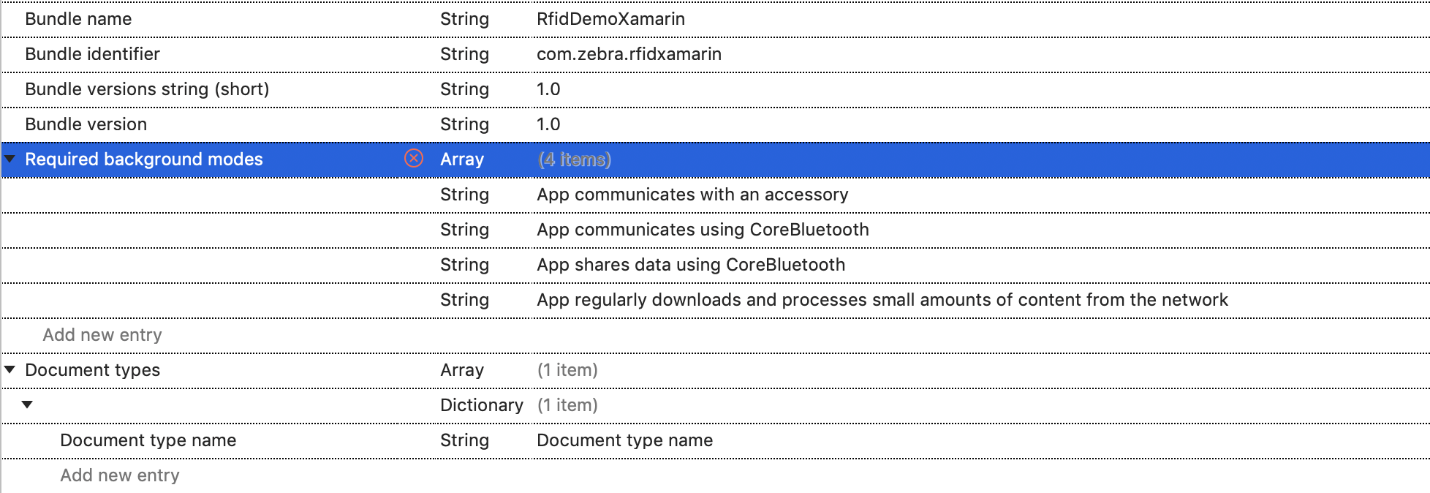
Add 'com.zebra.rfd8x00_easytext' String under 'Supported external accessory protocols' property.
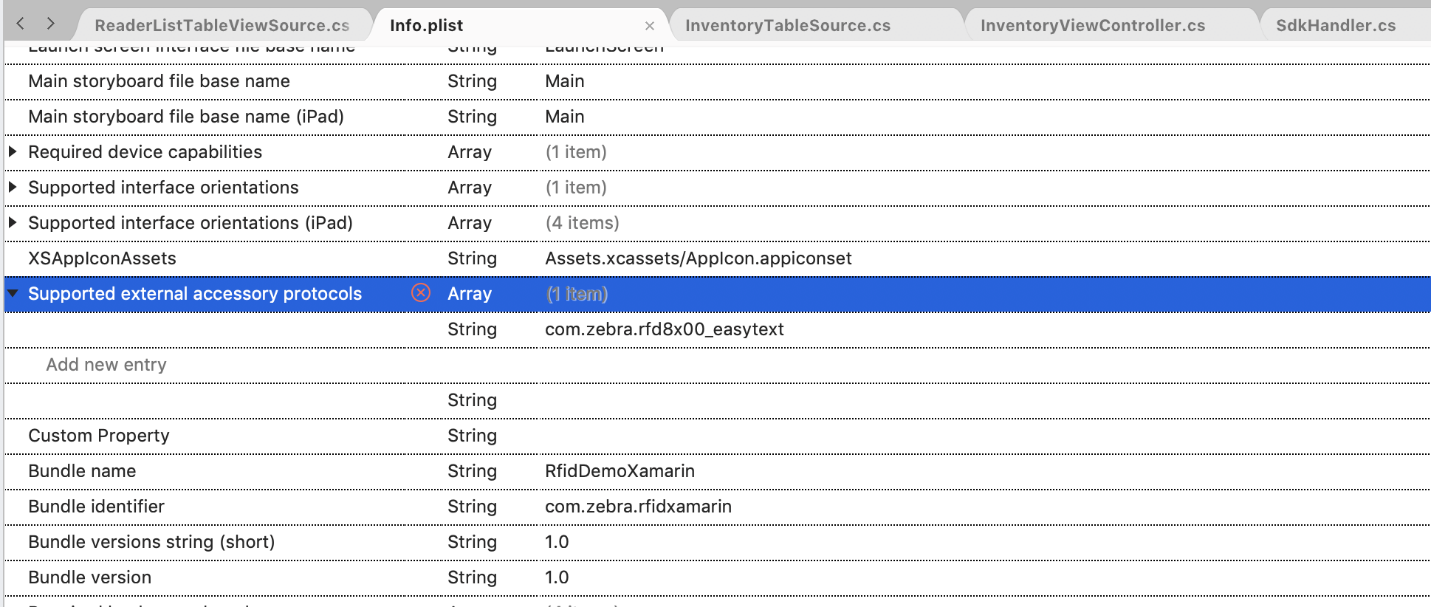
Wrapper APIs
Namespace
Import the RFID SDK namespace before making API calls.
using ZebraRfidSdk;
Query SDK Version
Version information could be queried as follows.
//Create an instance of the RfidSDK
RfidSdk rfidSdk = new RfidSdk();
//Get the version
string version = rfidSdk.Version;
Set Operation Mode
Set the operation mode of the reader.
//Create an instance of the Readers
Readers readers = rfidSDK.ReaderManager;
//Set Operation Mode
readers.SetOperationMode(OpMode.OPMODE_MFI);
Supports following operation modes,
- OPMODE_MFI
- OPMODE_BTLE
- OPMODE_ALL
Get Available Reader List
Query paired device list as follows. Reader must be paired with the iOS device via Bluetooth before query action.
//Get available readers list
List readerList = readers.GetReaders();
Connect/Disconnect RFID Reader
Connect to the first available reader.
//Connect to a given reader
readerList[0].Connect();
Disconnected from the connected reader.
//Connect to a given reader
readerList[0].Disconnect();
Start/Stop Inventory
RFID tag reading can be started as follows. Once started, tags in the range will be read continuously.
//Start reading available RFID tags
reader.Actions.Inventory.Start();
RFID tag reading cycle can be terminated as follows.
//Stop reading RFID tags
reader.Actions.Inventory.Stop();
Start/Stop Tag Locate
Tag locating can be started as follows.
//Locate tags
reader.Actions.TagLocate.Start("");
Stop locating tags.
//Stop locating tags
reader.Actions.TagLocate.Stop();
Start/Stop Trigger Configuration
Set Start Trigger Configuration to the reader.
//Set configurations
StartTriggerConfiguration configuration = new StartTriggerConfiguration();
configuration.RepeatMonitoring = true;
configuration.StartDelay = 1;
configuration.StartOnHandheldTrigger = true;
//Set start trigger configurations
reader.Configuration.StartTriggerConfiguration = configuration;
Available Start Trigger configurations;
- RepeatMonitoring : bool
- StartOnHandheldTrigger : bool
- StartDelay : int
- TriggerType : TriggerType
Set Stop Trigger Configuration to the reader.
//Set configurations
StopTriggerConfiguration configuration = new StopTriggerConfiguration();
configuration.StopOnAccessCount = true;
configuration.StopInventoryCount = 1;
configuration.StopAccessCount = 1;
//Set start trigger configurations
reader.Configuration.StopTriggerConfiguration = configuration;
Available options for Stop Trigger Configuration;
- StopOnAccessCount : bool
- StopOnHandheldTrigger : bool
- StopOnInventoryCount : bool
- StopOnTagCount : bool
- StopOnTimeOut : bool
- DurationMilliSecond : int
- StopAccessCount : int
- StopInventoryCount : int
- StopTagCount : int
- StopTimeOut : int
Set Batch Mode Configuration
Batch Mode configurations can be applied as follows. Supported values for Batch Mode are Auto, Disable and Enable.
//Set Batch mode
reader.Configuration.BatchModeConfiguration = BatchMode.AUTO;
Set Unique Tag Report
Unique Tag Report can be applied as follows. Supported values are true or false.
//Set Unique Tag Report
reader.Configuration.UniqueTagReport = true;
Set Tag Report Configuration
Tag Report Configuration can be applied as follows.
//Set Tag Report Configurations
TagReportConfiguration configuration = new TagReportConfiguration();
configuration.ChannelIdx = true;
configuration.FirstSeenTime = true;
configuration.Pc = true;
configuration.Rssi = true;
reader.Configuration.TagReportConfiguration = configuration;
Available options for Tag Report Configuration;
- ChannelIdx : bool
- FirstSeenTime : bool
- LastSeenTime : bool
- Pc : bool
- Phase : bool
- Rssi : bool
- TagSeenCount : bool
Set Regulatory Configuration
Regulatory Configuration can be applied as follows.
//Set Regulatory Configurations
RegulatoryConfig regulatoryConfig = new RegulatoryConfig();
regulatoryConfig.HoppingOn = true;
reader.Configuration.RegulatoryConfig = regulatoryConfig;
Available options for Regulatory Config;
- HoppingOn : bool
- EnableChannels : object[]
- HoppingConfig : HoppingConfig
- RegionCode : string
Set Singulation Configuration
Singulation Control configurations can be applied as follows.
//Set Singulation Configurations
SingulationControl singulationControl = new SingulationControl();
singulationControl.SelectedFlag = SLFlag.SLFLAG_ALL;
singulationControl.Session = Session.SESSION_S0;
singulationControl.State = InventoryState.INVENTORYSTATE_A;
singulationControl.TagPopulation = 200;
reader.Configuration.Antennas.singulationControl = singulationControl;
Available options for Singulation Configuration.
- SelectedFlag : SLFlag
- Session : Session
- State : InventoryState
- TagPopulation : int
Set Device Mode
Set device mode of the reader.
//Set Device Mode
reader.Configuration.SetDeviceMode(DeviceMode.RFID);
Available Device Modes;
- RFID
- BARCODE
Events
Activity Events
Appeared
This event is triggered when the readers appeared.
// readerInfo – Object containing information of the appeared reader
readers.Appeared += (readerInfo) =>
{
//Action
};
Disappeared
This event triggers when the readers disappeared.
// readerID - ID of the disappeared reader.
readers.Disappeared += (readerID) =>
{
//Action
};
Note: Appeared and Disappeared events are not supported in RFID SDK for Xamarin.Android. This causes some incorrect behaviors and incorrect tag data retrievals when the scanner goes out and comes back to the range. It is recommended not to use the reader in ‘out of range’ scenario with RFID SDK for Xamarin.Android.
Connected
This event triggers when the readers are connected.
// readerInfo - provides information of the reader connected
readers.Connected += (readerInfo) =>
{
//Action
};
Disconnected
This event triggers when the readers are disconnected.
// readerID - readerID of the reader disconnected
readers.Disconnected += (readerID) =>
{
//Action
};
TagDataEvent
This event triggers when tag data is received.
// tagdata - provides information of the tag read by the reader
reader.TagDataEvent += (tagData) =>
{
//Action
};
ProximityPercent
This event will trigger when tags are nearby.
// proximityPercent - provides proximity information of the tag from the reader
reader.ProximityPercent += (proximityPercent) =>
{
//Action
};
Action Status Events
Following events can be registered to get RFID reader related information.
- OperationEndSummary
- Temperature
- Power
- Database
- Radio
- OperationStop
- OperationBatchmode